세션 키 암호화
1 개념
가) 비대칭 암호화는 대칭 암호화보다 1000배 느림
나) 메시지는 대칭 키로 암호화하고 이 대칭 키가 수신자의 공개키로 암호화됨
다) 암호화된 공개 키는 메시지에 덧붙여서 수신자에게 전달됨
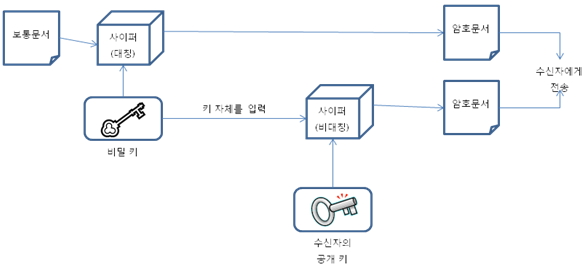
2 예제
import java.io.UnsupportedEncodingException; import java.security.InvalidKeyException; import java.security.Key; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException; import javax.crypto.Cipher; import javax.crypto.IllegalBlockSizeException; import javax.crypto.KeyGenerator; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec;
public class SimpleRSAExample { public static void main(String[] args) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException, UnsupportedEncodingException { String plainText = "iloveyou"; // 대칭 키 생성 KeyGenerator keyGenerator = KeyGenerator.getInstance("Blowfish"); keyGenerator.init(128); Key blowfishKey = keyGenerator.generateKey(); Cipher blowfishCipher = Cipher.getInstance("Blowfish/ECB/PKCS5padding"); blowfishCipher.init(Cipher.ENCRYPT_MODE, blowfishKey); byte[] ciphertext = blowfishCipher.doFinal(plainText.getBytes()); System.out.println("암호화된 문서 : " + ciphertext); // RSA 키 쌍 생성 KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA"); keyPairGenerator.initialize(1024); KeyPair keyPair = keyPairGenerator.genKeyPair(); Cipher rsaCipher = Cipher.getInstance("RSA/ECB/PKCS1Padding"); rsaCipher.init(Cipher.ENCRYPT_MODE, keyPair.getPublic()); //대칭 키의 바이트 획득 byte[] blowfishKeyBytes = blowfishKey.getEncoded(); // 대칭 키 바이트를 RSA 개인키로 암호화 byte[] cipherKeyBytes = rsaCipher.doFinal(blowfishKeyBytes); // 수신측에서 동작한다고 가정 // 암호화된 문서와 암호화된 대칭키가 전달된다고 가정 // 수신자의 공개키로 키 복호화 rsaCipher.init(Cipher.DECRYPT_MODE, keyPair.getPrivate()); byte[] decryptedKeyBytes = rsaCipher.doFinal(cipherKeyBytes); SecretKey newBlowfishKey = new SecretKeySpec(decryptedKeyBytes, "Blowfish"); // 복호화된 대칭키로 전달된 암호문 복호화 blowfishCipher.init(Cipher.DECRYPT_MODE, newBlowfishKey); byte[] decryptedText = blowfishCipher.doFinal(ciphertext); System.out.println("복호화된 문서 : " + new String(decryptedText)); }
} |